RESTful CRUD API using Nodejs,express and mysql : Part 3 – Implementing CRUD
In the last post, we looked at how to integrate the MySQL database using ORM (Sequelize). In this post, we will further enhance the API which we created by adding full CRUD functionality.
Let’s start by adding the POST method for adding a new Employee.
Add the following code employee.controller.js file.
const post = async (req,res) => {
var employee = req.body;
const createdEmployee = await Employee.create(employee);
res.statusCode = 201;
res.json(createdEmployee);
}
and also add the ‘post’ method to exports.
In this code, we are extracting the JSON payload having employee data from the body of the request and passing it to create a method provided by sequelize (read more here on Model instances)to save it in the database.
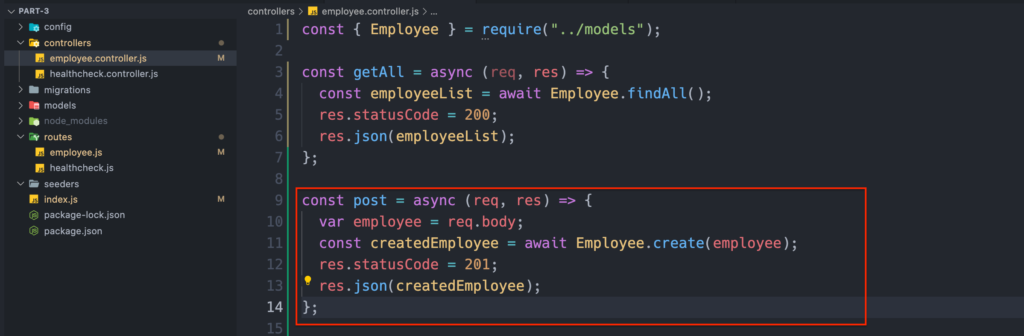
To make this work we need to add this post method to the employee router.
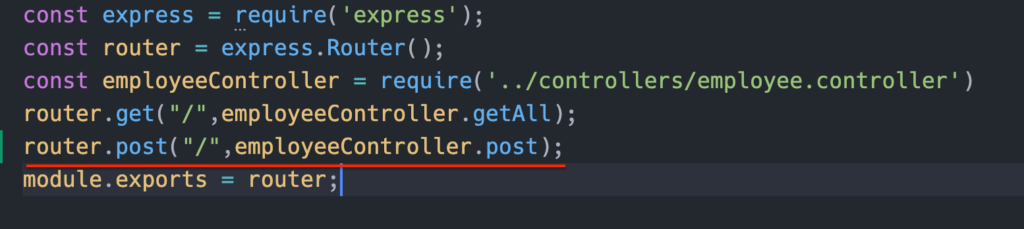
Now, let’s run the server and try to post an employee request using postman.
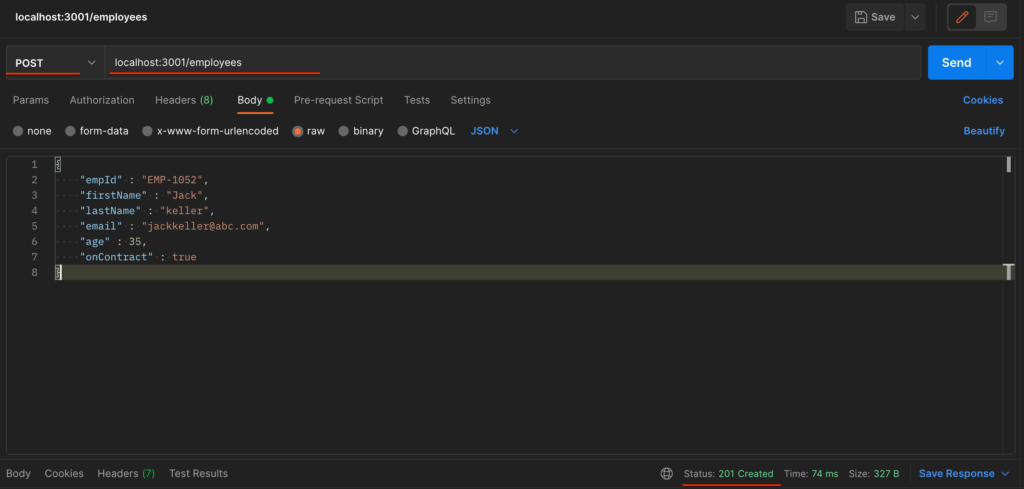
You will notice that the request is successful with 201 Created responses and the console also shows successful query executed. But below is what is added to the database i.e. all the values are null.

The reason being we are missing one important middleware for processing JSON request bodies. Let’s add that by adding the below the line in index.js.
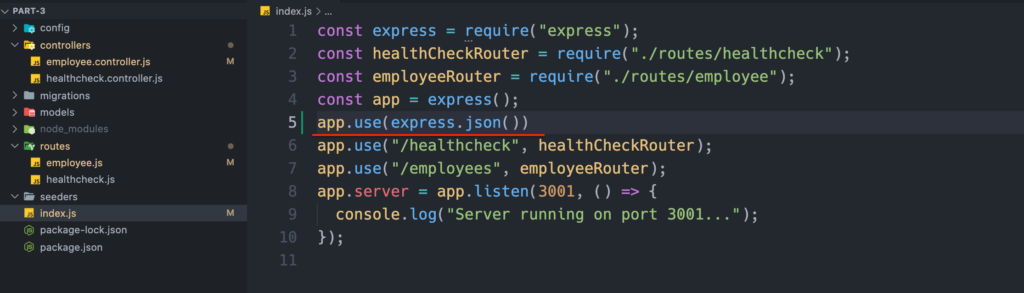
This is a built-in middleware in the express and is used to parse the JSON data coming in the request body. Without this express cannot interpret the JSON payload in request. Let’s try to run our server and send requests again. This time it works.

Now we have our POST requests working. Let’s add a PUT request next i.e. PUT /employees/1
PUT as you know is used to update a resource or entity on the server and hence we have to provide a specific resource id in URL.
const put = async (req, res) => {
var id = req.params.id;
var employee = req.body;
var updateCondition = {where : {id : id}}
await Employee.update(employee,updateCondition);
res.status(204).end();
};
The code above is similar to POST with few differences.
- We need to extract the id from request parameters (i.e. req.params.id)
- As we need to update an existing entity in database sequelize provides update method on the model which takes a form of JSON query specified above in ‘updateCondition’.
Similarly, let’s add the DELETE request i.e. DELETE /employees/1 by adding the below code.
const remove = async (req,res) => {
var id = req.params.id;
var deleteCondition = {where : {id : id}};
await Employee.destroy(deleteCondition);
res.status(204).end();
}
This is again self-explanatory. The only major difference is the ‘destroy’ method used with only the delete condition.
Make sure to add correct routes as shown below for PUT and DELETE.
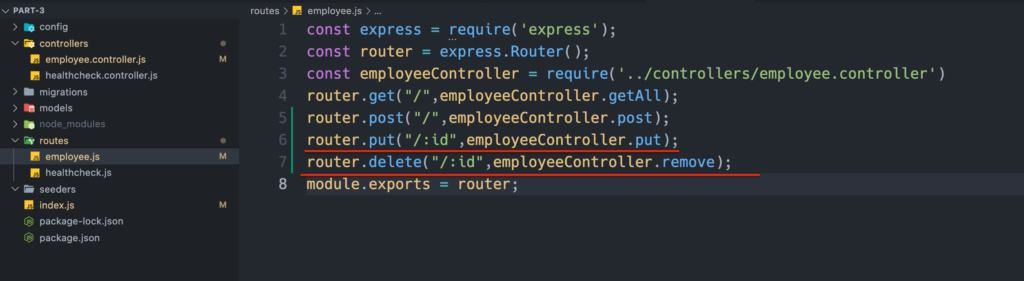
Go ahead and test this out by running the server (i.e. npm start) and using a client like Postman.
Please note that as this code is more focused on the basic stuff this code does not implement any of the error checking and exception handling which is required for a production code. We might get there in future posts but for now, this is a full-fledged CRUD API.
All of the code for this post is available here.
If you are looking into a deep dive into nodejs i.e. basically going from beginner to advanced level do checkout this pluralsight skills path.
In the next post, I would introduce how to do unit testing for an API like this.